JS Tools
class: center, middle .title[ Front-end training # JS Tools ] --- # What is NodeJS ? Node.js is an open-source, cross-platform runtime environment for developing server-side Web applications. Although Node.js is not a JavaScript framework, many of its basic modules are written in JavaScript, and developers can write new modules in JavaScript. The runtime environment interprets JavaScript using Google's V8 JavaScript engine. Node.js has an event-driven architecture capable of asynchronous I/O. These design choices aim to optimize throughput and scalability in Web applications with many input/output operations, as well as for real-time Web applications (e.g., real-time communication programs and browser games). .center[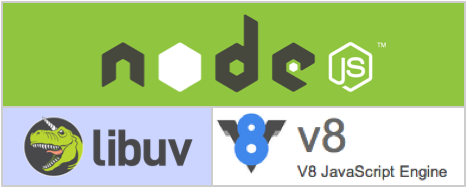] --- # NodeJS installation If you're using OS X or Windows, the best way to install Node.js is to use one of the installers from http://nodejs.org. If you're using Linux, you can use the installer, or you can check NodeSource's binary distributions (https://downloads.nodesource.com/) to see whether or not there's a more recent version that works with your system. Test: Run **node -v**. The version should be higher than v4.4.7. --- # Tools Classification - One purpose tools (cssmin, imagemin, uglify) - Client-side package managers (NPM, Bower) - Package Bundlers (Browserify, Webpack) - Task runners (Grunt, Gulp) - Scaffolders (Yeoman) --- # Client-side package management What for? - Install dependencies (like jQuery plugins or so) - Keep project vendor dependencies up-to-date - Easily build your application --- # NPM Initialise an NPM package. Creates a package.json file for you: ```bash npm init ``` Most common commands: ```bash npm install {package name} sudo npm install {package name} -g #install package globally npm install {package name} --save-dev #write module as a devDependency to package.json npm uninstall {package name} npm cache clean npm list npm prune #cleans node_modules of those packages which are not listed in package.json ``` .center[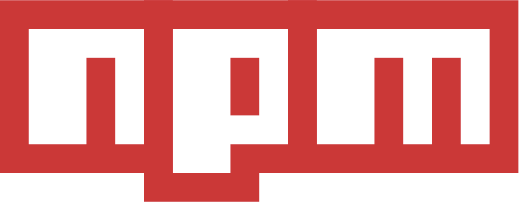] --- # Bower http://bower.io/ ```bash sudo npm install -g bower bower install bower install jquery ``` .center[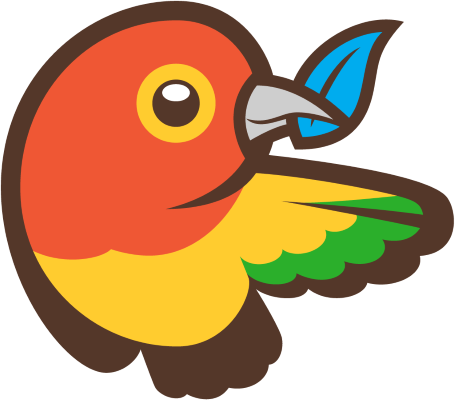] --- # Package Bundlers What for? - Bind up all of your modules and dependencies .center[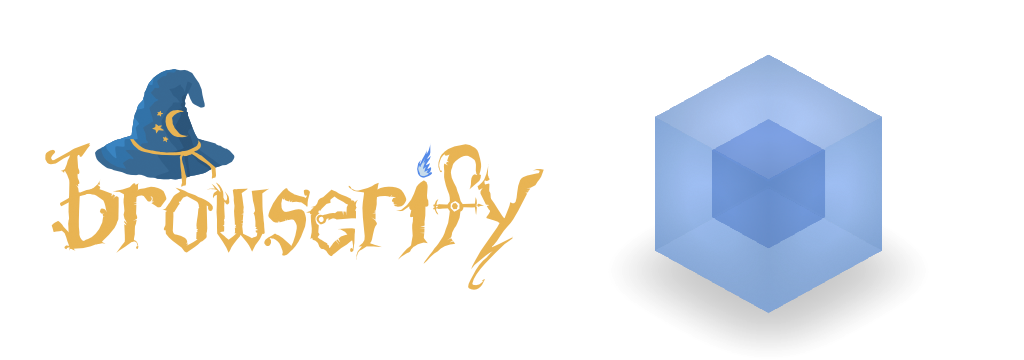] --- # Browserify https://www.npmjs.org/package/browserify Browserify lets you require('modules') in the browser by bundling up all of your dependencies. ### install ```bash npm install -g browserify ``` ### example ```javascript // main.js var foo = require('./foo.js'); var bar = require('../lib/bar.js'); var gamma = require('gamma'); var elem = document.getElementById('result'); var x = foo(100) + bar('baz'); elem.textContent = gamma(x); ``` ### bundle ```bash browserify main.js --output bundle.js ``` --- # Webpack ### install ```bash npm install webpack -g ``` ### example config file ```javascript // webpack.config.js module.exports = { entry: "./entry.js", output: { path: __dirname, filename: "bundle.js" }, module: { loaders: [ { test: /\.css$/, loader: "style!css" } ] } }; ``` ### run bundler ```bash webpack ``` --- # Build Systems (Task Runners) What for? - Automate routine tasks (linting, testing, compilation, etc.) - watch your changes appear in browser on the fly while development - etc., etc., etc. ... .center[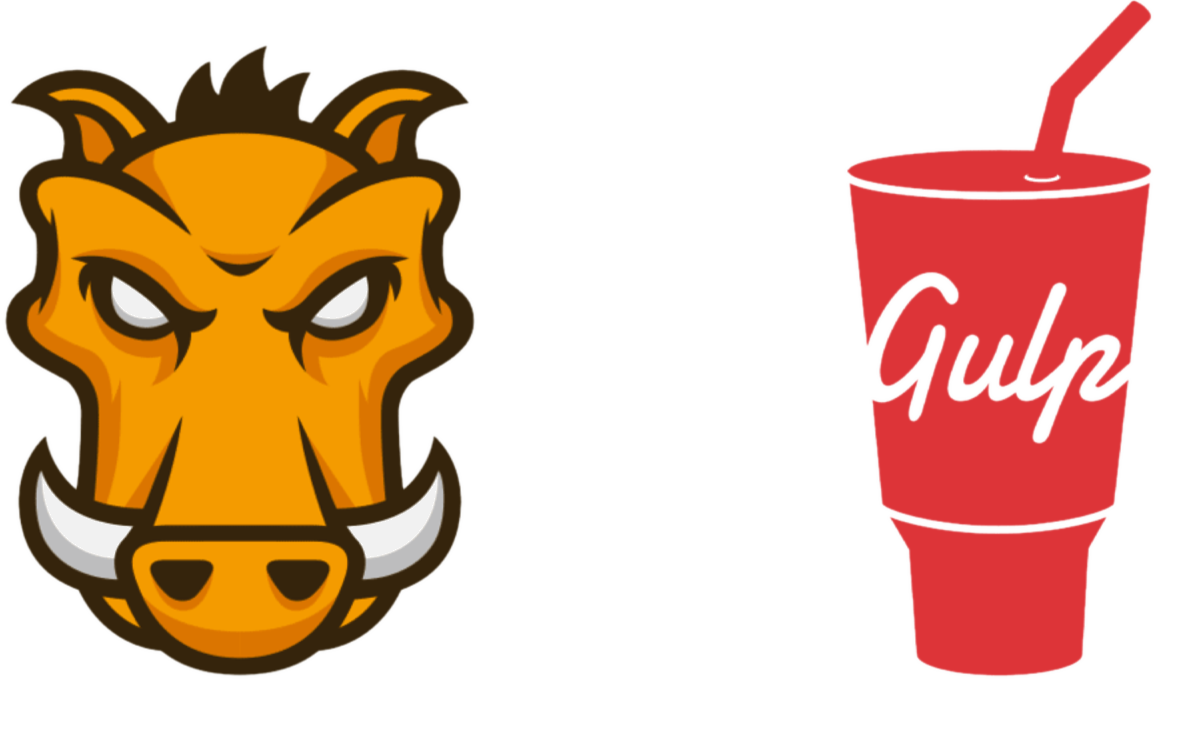] --- # Grunt Javascript task runner http://gruntjs.com/ ```bash sudo npm install -g grunt-cli npm install grunt grunt init #creates Gruntfile.js grunt {task}:{subtask} ``` ```js // Gruntfile.js module.exports = function(grunt) { grunt.initConfig({ // watch for file changes watch: { sass: { files: '**/*.scss', tasks: ['sass'] // anytime .scss files are changed - run sass:dev task } } }); // register tasks to be called from CLI grunt.registerTask('default', ['sass']); }; ``` --- # Gulp http://gulpjs.com/ Uses streams, therefore performance is better that of Grunt. Less configurations, more javascript code. ```bash sudo npm install -g gulp gulp build_scripts #custom task name ``` ```js // gulpfile.js var gulp = require('gulp'); var sass = require('gulp-sass'); gulp.task('sass', function () { // gulp.task registers task to be called from CLI gulp.src('./scss/*.scss') // gulp.src reads file into streams .pipe(sass()) // pipe any plugin/s .pipe(gulp.dest('./css')); // gulp.dest writes files to provided destination }); // gulp.task('default', 'sass'); ``` --- # Scaffolding What for? - Get the specific setup up-and-running in no time - Easier usage of best practices --- # Yeoman Yeoman helps you to kickstart new projects, prescribing best practices and tools to help you stay productive. - http://yeoman.io/ ```bash sudo npm install -g yo sudo npm install -g generator-angular yo angular {my_awesome_app_name} ``` .center[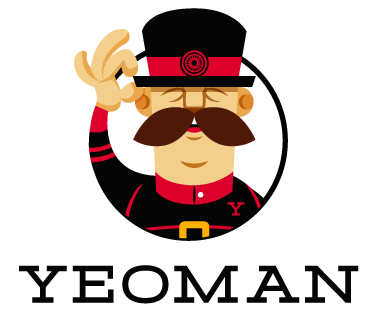]