AngularJS
class: center, middle .title[ 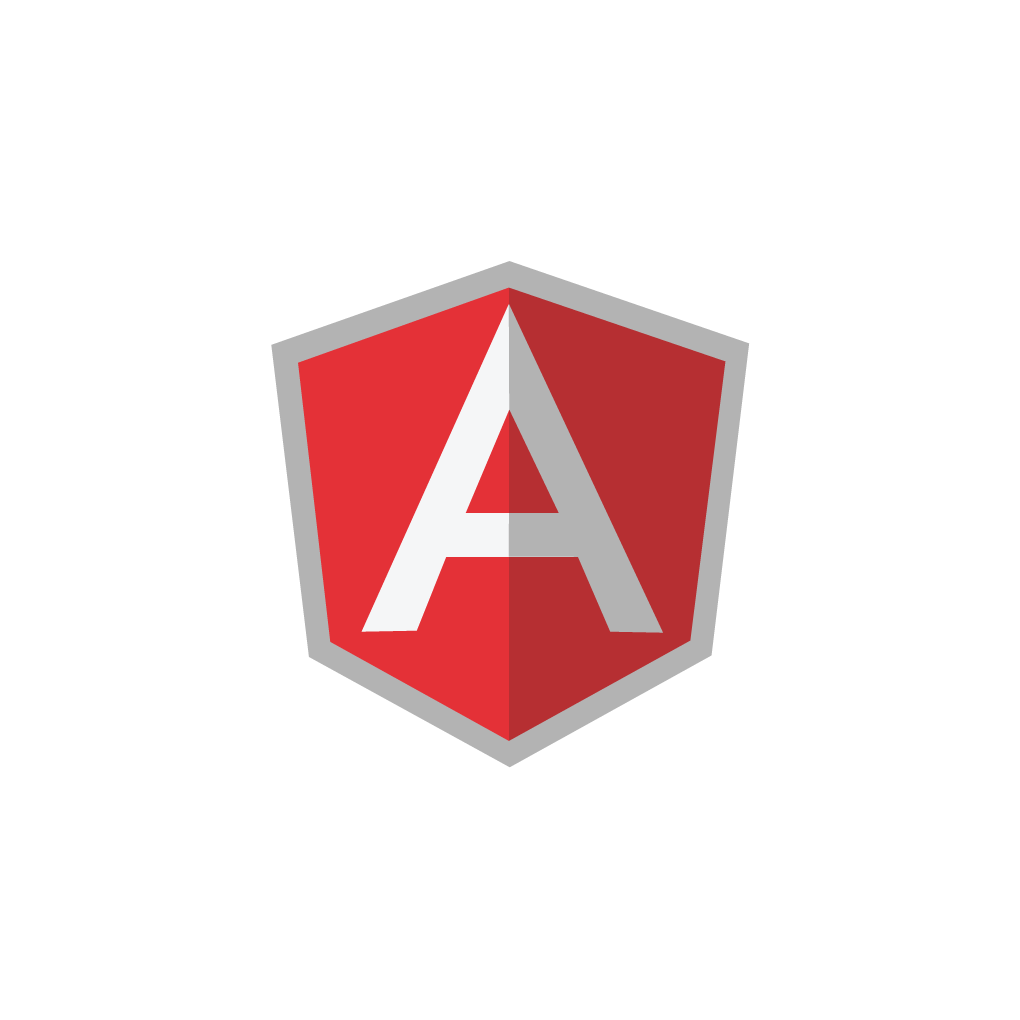 ] --- class: center, middle 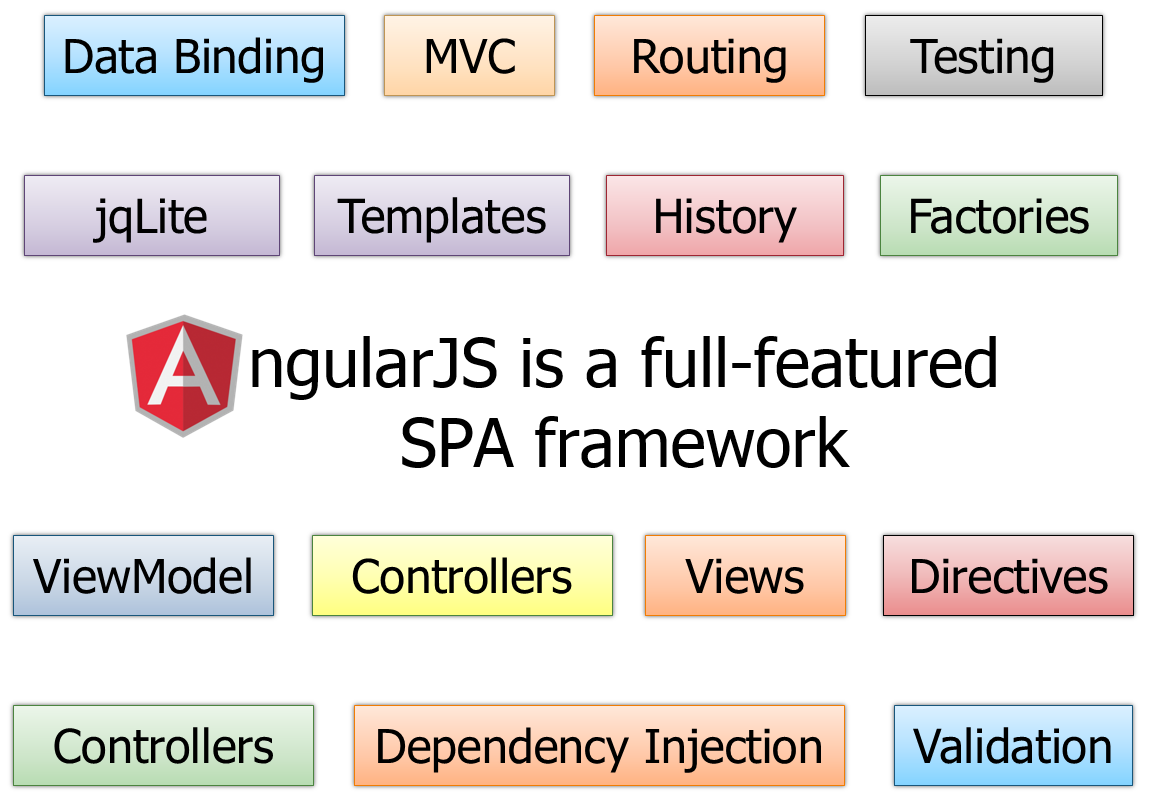 --- class: center middle .title[ # Simple App - [Demo](examples/simple-app.html) ] .demo[
] --- class: center, middle .title[ # Simple App - Code ] .left[ ## Angular module ```js angular.module('simpleApp',[]) .controller('Ctrl', function ( $scope ) { $scope.students = [ ... ]; }); ``` ] .left[ ## HTML markup ```html
Found: {{ query.length ? filtered.length : 0 }}
{{ name }}
``` ] --- class: center middle .title[ # How it works...? ## !@#$%^%$& ] 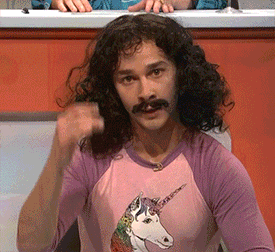 --- class: center, middle .title[ # B U T ... ] --- class: center, middle .title[ # Simple App - Code ] .left[ ## Angular module ```js angular.module('simpleApp',[]) .controller('Ctrl', function ( $scope ) { $scope.students = [ ... ]; }); ``` ] .left[ ## HTML markup ```html
Found: {{ query.length ? filtered.length : 0 }}
*
{{ name }}
``` ] --- class: center middle .title[ # Simple App Broken :(- [Demo](examples/simple-app-not-working.html) ] .demo[
] --- class: center, middle .title[ 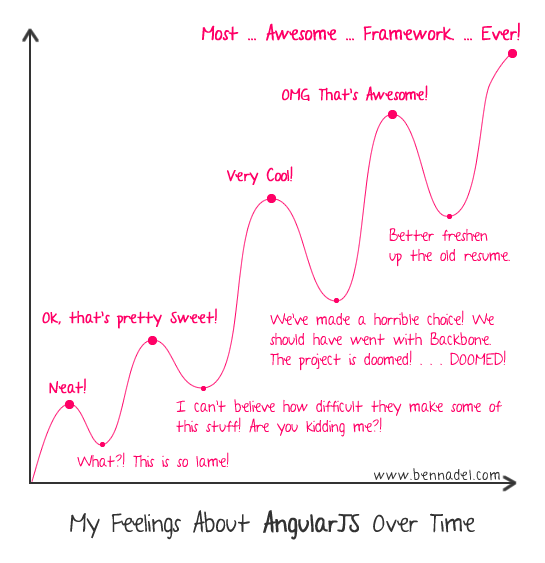 ] --- class: center middle .title[ #Concepts ] .left[ - ##Scope - ##Data Binding - ##Dependency Injection - ##Directive - ##Controller - ##Service ] --- class: center middle .title[ #Scope ] .left[ - ###Scope is an object that refers to the application model. - ###It is an execution context for expressions. - ###Scopes are arranged in hierarchical structure which mimic the DOM structure of the application. - ###Scopes can watch expressions and propagate events. ] --- class: center middle .title[ #Scope Characteristics ] .left[ - ###Scopes provide APIs (***$watch***) to observe model mutations. - ###Scopes provide APIs (***$apply***) to propagate any model changes through the system into the view from outside of the "Angular realm" (controllers, services, Angular event handlers). - ###Scopes provide APIs (***$on***, ***$emit***, ***$broadcast***) for dispatching/subscribing custom events - ###Scopes can be nested to limit access to the properties of application components while providing access to shared model properties. - ###Scopes provide context against which ***expressions*** are evaluated. ] --- class: center middle .title[ # Event Loop ] 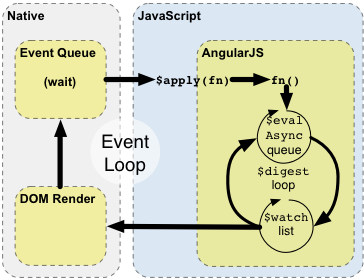 --- class: center middle .title[ #Data Binding ] .left[ - ###Any changes to the view are immediately reflected in the model, and any changes in the model are propagated to the view. - ###The model is the single-source-of-truth for the application state, greatly simplifying the programming model for the developer. - ###You can think of the view as simply an instant projection of your model. ] --- class: center middle 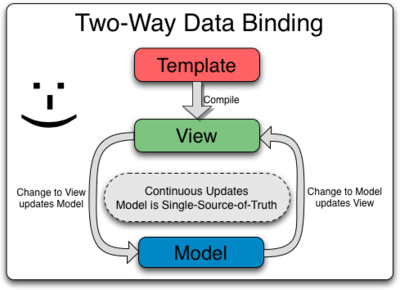 --- class: center middle .title[ #Dependency Injection ] ##There are only three ways a component (object or function) can get a hold of its dependencies: .left[ - ###The component can create the dependency, typically using the ***new*** operator. - ###The component can look up the dependency, by referring to a global variable. - ###The component can have the dependency passed to it where it is needed. ] --- class: center middle .title[ #Dependency Annotation ] .left[ - ###Using the inline array annotation (preferred) ```js someModule.controller('MyController', ['$scope', 'greeter', function($scope, greeter) { // ... }]); ``` - ###Using the $inject property annotation ```js var MyController = function($scope, greeter) { // ... } MyController.$inject = ['$scope', 'greeter']; someModule.controller('MyController', MyController); ``` - ###Implicitly from the function parameter names (has caveats) ```js someModule.controller('MyController', function($scope, greeter) { // ... }); ``` ] --- class: center middle .title[ #Directive ] .left[ ```js myModule.directive('directiveName', function factory(injectables) { var directiveDefinitionObject = { priority: 0, template: '
', // templateUrl: 'directive.html', transclude: false, restrict: 'E',// 'A', 'C', 'M' scope: false, // true, {} controller: function($scope, $element, $attrs, $transclude, otherInjectables) { ... }, controllerAs: 'stringIdentifier', bindToController: false, link: function postLink( scope, iElement, iAttrs, controller ) { ... } }; return directiveDefinitionObject; }); ``` ```html
``` ] --- class: center middle .title[ #Practice ] .middle[ .img-wrap-70[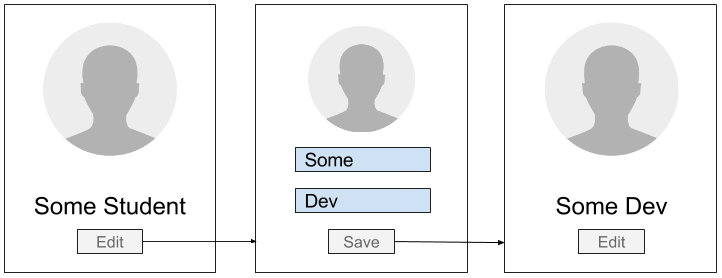] ] .center[ ##Create angular directive to display/edit student info in card view. ([ref1](practice/index.html), [ref2](https://plnkr.co/edit/ZejRPicd7UjDD3UIso9Y?p=preview)) ] .left[ - create js file called studentname-studentsurname.js (e.g. john-doe.js) - create angular module called 'studentname.studentsurname' (e.g. 'john.doe') - create angular directive that can be used as element ```
``` (e.g. ```
```) ] --- # Related resources - [AngularJS API Reference](https://docs.angularjs.org/api) - [Creating AngularJS Custom Directives](http://weblogs.asp.net/dwahlin/creating-custom-angularjs-directives-part-i-the-fundamentals) - [Angular 1 Style Guide](https://github.com/johnpapa/angular-styleguide/blob/master/a1/README.md)